Meshes
The two-dimensional embedded delta sets (EmbeddedDeltaSet2D
) in CombinatorialSpaces can be converted to and from mesh objects (Mesh
) in Meshes.jl. This is useful for interoperation with packages in the JuliaGeometry ecosystem.
Visualizing embedded delta sets
The following example shows how to import a mesh from an OBJ file, convert it into an embedded delta set, and render it as a 3D mesh using CairoMakie.
using FileIO, CairoMakie, CombinatorialSpaces
set_theme!(resolution=(800, 400))
catmesh = FileIO.load(File{format"OBJ"}(download(
"https://github.com/JuliaPlots/Makie.jl/raw/master/assets/cat.obj")))
catmesh_dset = EmbeddedDeltaSet2D(catmesh)
mesh(catmesh_dset, shading=false)
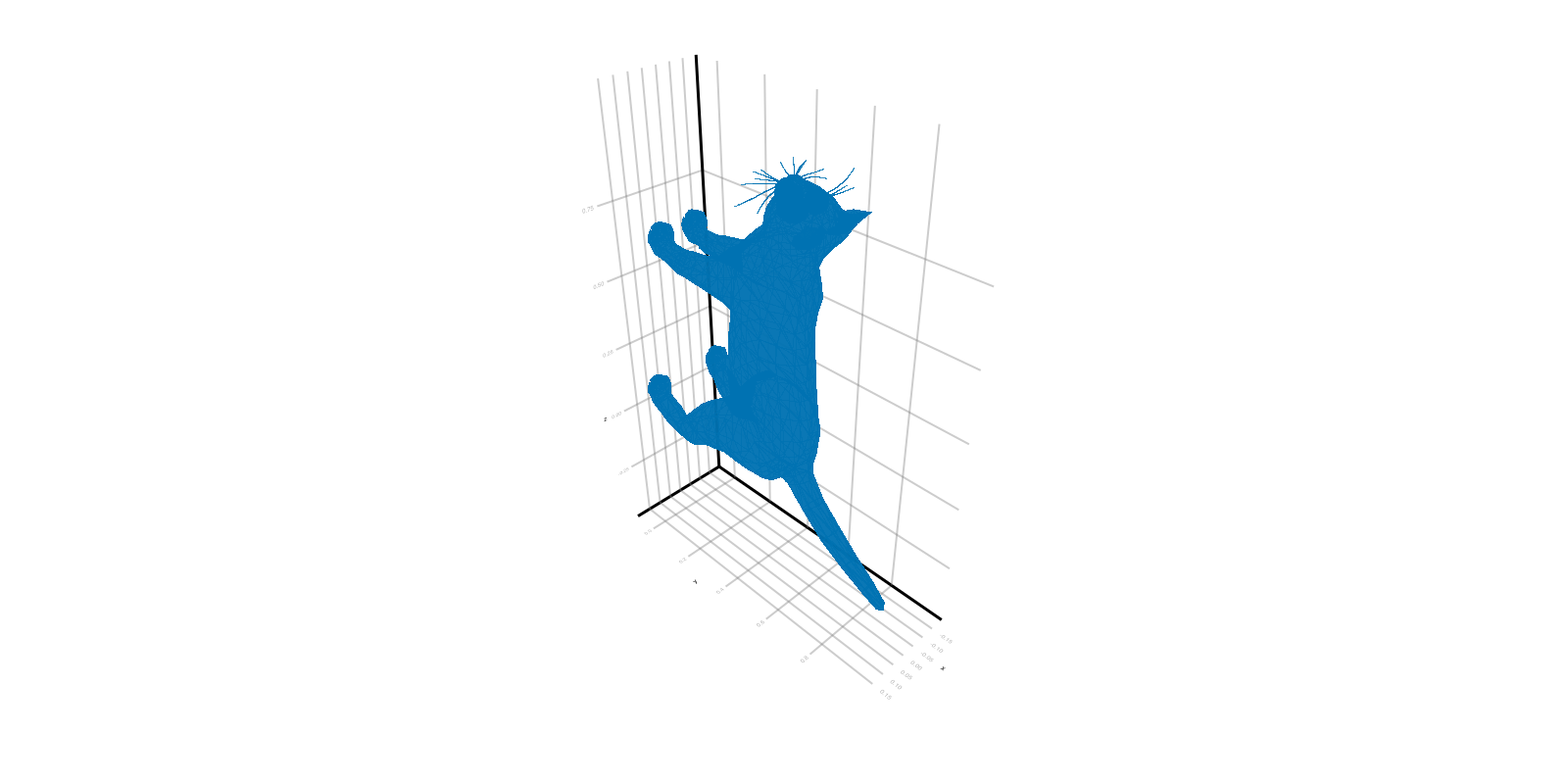
Alterntively, the embedded delta set can be visualized as a wireframe:
wireframe(catmesh_dset)

We can also construct and plot the dual complex for this mesh:
dual = EmbeddedDeltaDualComplex2D{Bool, Float32, Point{3,Float32}}(catmesh_dset)
subdivide_duals!(dual, Barycenter())
wireframe(dual)

API docs
CombinatorialSpaces.Meshes.loadmesh
— Methodloadmesh(s::Icosphere)
Load in a icosphere mesh.
CombinatorialSpaces.Meshes.loadmesh
— Methodloadmesh(s::Point_Map)
Load in a point map describing the connectivity of the toroidal mesh.
CombinatorialSpaces.Meshes.loadmesh
— Methodloadmesh(s::Rectangle_30x10)
Load in a rectangular mesh.
CombinatorialSpaces.Meshes.loadmesh
— Methodloadmesh(s::Torus_30x10)
Load in a toroidal mesh.
CombinatorialSpaces.Meshes.makeSphere
— MethodmakeSphere(minLat, maxLat, dLat, minLong, maxLong, dLong, radius)
Construct a spherical mesh (inclusively) bounded by the given latitudes and longitudes, discretized at dLat and dLong intervals, at the given radius from Earth's center.
Note that this construction returns a UV-sphere. DEC simulations are more accurate on meshes with (near) equilateral triangles, such as the icospheres available through loadmesh
.
We say that:
- 90°N is 0
- 90°S is 180
- Prime Meridian is 0
- 10°W is 355
We say that:
- (x=0,y=0,z=0) is at the center of the sphere
- the x-axis points toward 0°,0°
- the y-axis points toward 90°E,0°
- the z-axis points toward the North Pole
References:
List of common coordinate transformations
Examples
# Regular octahedron.
julia> s, npi, spi = makeSphere(0, 180, 90, 0, 360, 90, 1)
# 72 points along the unit circle on the x-y plane.
julia> s, npi, spi = makeSphere(90, 90, 0, 0, 360, 5, 1)
# 72 points along the equator at 0km from Earth's surface.
julia> s, npi, spi = makeSphere(90, 90, 1, 0, 360, 5, 6371)
# TIE-GCM grid at 90km altitude (with no poles, i.e. a bulbous cylinder).
julia> s, npi, spi = makeSphere(5, 175, 5, 0, 360, 5, 6371+90)
# TIE-GCM grid at 90km altitude (with South pole, i.e. a bowl).
julia> s, npi, spi = makeSphere(5, 180, 5, 0, 360, 5, 6371+90)
# TIE-GCM grid at 90km altitude (with poles, i.e. a sphere).
julia> s, npi, spi = makeSphere(0, 180, 5, 0, 360, 5, 6371+90)
# The Northern hemisphere of the TIE-GCM grid at 90km altitude.
julia> s, npi, spi = makeSphere(0, 180, 5, 0, 360, 5, 6371+90)
CombinatorialSpaces.Meshes.triangulated_grid
— Methodfunction triangulated_grid(max_x, max_y, dx, dy, point_type)
Return a grid of (near) equilateral triangles.
Note that dx will be slightly less than what is given, since points are compressed to lie within max_x.
CombinatorialSpaces.MeshInterop
— ModuleInteroperation with mesh files.
This module enables delta sets to be imported from mesh files supported by MeshIO.jl and for delta sets to be converted to meshes, mainly for the purposes of plotting. Meshes are represented by the GeometryBasics.Mesh
type.